December 12, 2024 by Appler LABS
Embedded Dashboards - Apache Superset Embedding Flow and Guest Tokens
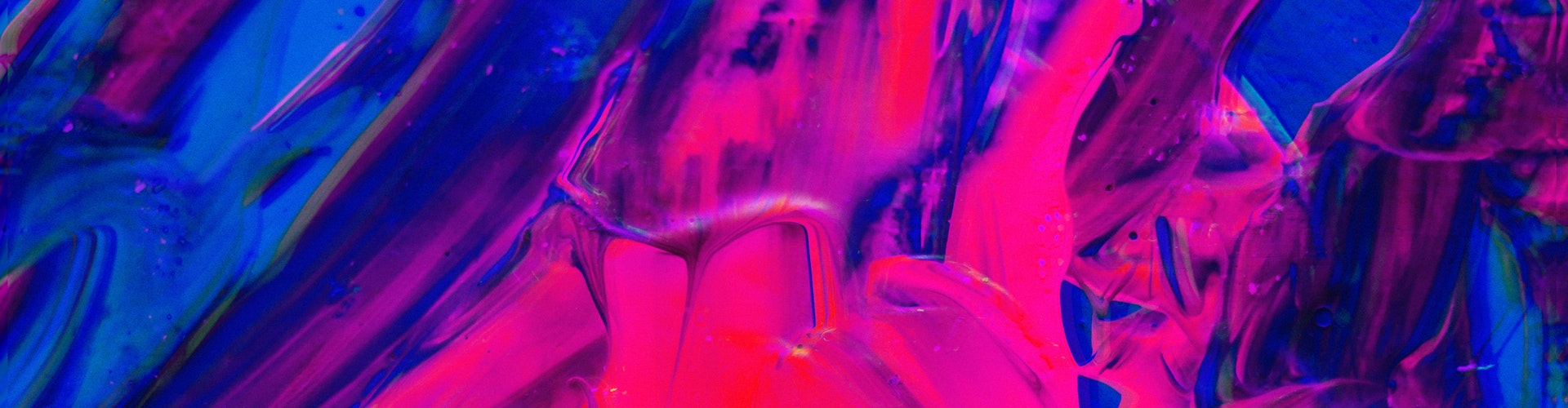
Embedded Dashboards: Apache Superset Embedding Flow and Guest Tokens
Embedding a dashboard in Apache Superset for a guest token flow with HTTPOnly
cookies and Talisman
Content Security Policy (CSP) enabled requires careful configuration to ensure security and functionality. Below, I’ll guide you through the steps and considerations for achieving this setup.
1. Understanding the Requirements
Guest Token Flow:
- A guest token is a temporary token issued to unauthenticated users to access specific dashboards or reports.
- This token should be short-lived and have limited permissions.
HTTPOnly Cookies:
HTTPOnly
cookies are used to prevent client-side scripts from accessing the cookie, reducing the risk of XSS attacks.
Talisman CSP:
- Talisman is a Flask extension that enforces Content Security Policy (CSP) to mitigate XSS and other attacks.
Embedding Dashboards:
- You want to embed dashboards in an iframe or external application while maintaining security.
2. Steps to Embed a Dashboard with Guest Token Flow
Step 1: Enable Guest Token Flow in Superset
Superset does not natively support guest tokens out of the box, but you can implement this by:
- Creating a custom authentication flow.
- Issuing a short-lived JWT (JSON Web Token) or API key for guest users.
- Restricting the token’s permissions to specific dashboards or datasets.
Step 2: Configure HTTPOnly Cookies
To use HTTPOnly
cookies for guest tokens:
Set the
HTTPOnly
flag when issuing the cookie in your authentication flow.from flask import make_response, current_app @app.route('/issue-guest-token') def issue_guest_token(): token = generate_guest_token() # Implement your token generation logic response = make_response("Guest token issued") response.set_cookie('guest_token', token, httponly=True, secure=True, samesite='Strict') return response
Ensure the cookie is sent with requests to Superset.
Step 3: Enable Talisman CSP
Talisman is already part of Superset’s security stack. To configure it for embedding:
Modify the
Talisman
configuration in your Supersetconfig.py
:from flask_talisman import Talisman # Allow embedding in specific domains csp = { 'default-src': [ '\'self\'', 'https://your-external-domain.com', # Allow embedding from this domain ], 'script-src': [ '\'self\'', 'https://your-external-domain.com', ], 'frame-ancestors': [ '\'self\'', 'https://your-external-domain.com', ], } Talisman(app, content_security_policy=csp)
Ensure the
frame-ancestors
directive allows embedding from the desired domain.
Step 4: Embed the Dashboard
To embed the dashboard in an iframe:
Use the guest token to authenticate the user.
Embed the dashboard in an iframe with the appropriate URL.
<iframe src="https://your-superset-domain.com/superset/dashboard/1/?guest_token=YOUR_GUEST_TOKEN" width="100%" height="600px" frameborder="0" ></iframe>
Ensure the guest token is passed as a query parameter or in the request headers.
Step 5: Secure the Guest Token
To secure the guest token:
- Use short-lived tokens with a short expiration time.
- Restrict the token’s permissions to specific dashboards or datasets.
- Validate the token on the server side before granting access.
3. Considerations and Best Practices
Security Considerations
- Token Expiry: Ensure the guest token has a short expiration time (e.g., 5-10 minutes).
- Token Scope: Limit the token’s permissions to specific dashboards or datasets.
- CSRF Protection: Use anti-CSRF tokens or mechanisms to prevent CSRF attacks.
- HTTPS: Always use HTTPS to encrypt communication between the client and server.
Performance Considerations
- Token Refresh: Implement a mechanism to refresh the guest token if the user is active.
- Caching: Use caching for frequently accessed dashboards to reduce server load.
User Experience
- Error Handling: Provide clear error messages if the guest token is invalid or expired.
- UI/UX: Ensure the embedded dashboard is responsive and works well in the iframe.
4. Example Workflow
Guest Token Issuance:
- A user requests access to a dashboard.
- The server issues a guest token with limited permissions and a short expiration time.
Embedding the Dashboard:
- The guest token is passed to the iframe URL.
- The iframe loads the dashboard from Superset.
Token Validation:
- Superset validates the guest token and grants access to the dashboard.
Token Expiry:
- If the token expires, the user is redirected to a login page or shown an error message.
5. Sample Code
Flask Route for Guest Token Issuance
@app.route('/issue-guest-token')
def issue_guest_token():
token = generate_guest_token() # Implement your token generation logic
response = make_response("Guest token issued")
response.set_cookie('guest_token', token, httponly=True, secure=True, samesite='Strict')
return response
Embedding the Dashboard
<iframe
src="https://your-superset-domain.com/superset/dashboard/1/?guest_token=YOUR_GUEST_TOKEN"
width="100%"
height="600px"
frameborder="0"
></iframe>
Talisman Configuration
from flask_talisman import Talisman
csp = {
'default-src': [
'\'self\'',
'https://your-external-domain.com',
],
'script-src': [
'\'self\'',
'https://your-external-domain.com',
],
'frame-ancestors': [
'\'self\'',
'https://your-external-domain.com',
],
}
Talisman(app, content_security_policy=csp)
6. Conclusion
Embedding a dashboard in Apache Superset for a guest token flow with HTTPOnly
cookies and Talisman
CSP enabled is achievable with careful configuration. By following the steps above, you can securely embed dashboards while maintaining strong security practices. Ensure that your guest tokens are short-lived, permissions are restricted, and CSP is configured to allow embedding from trusted domains.