October 13, 2024 by Appler LABS
Flask backend and a Next frontend together
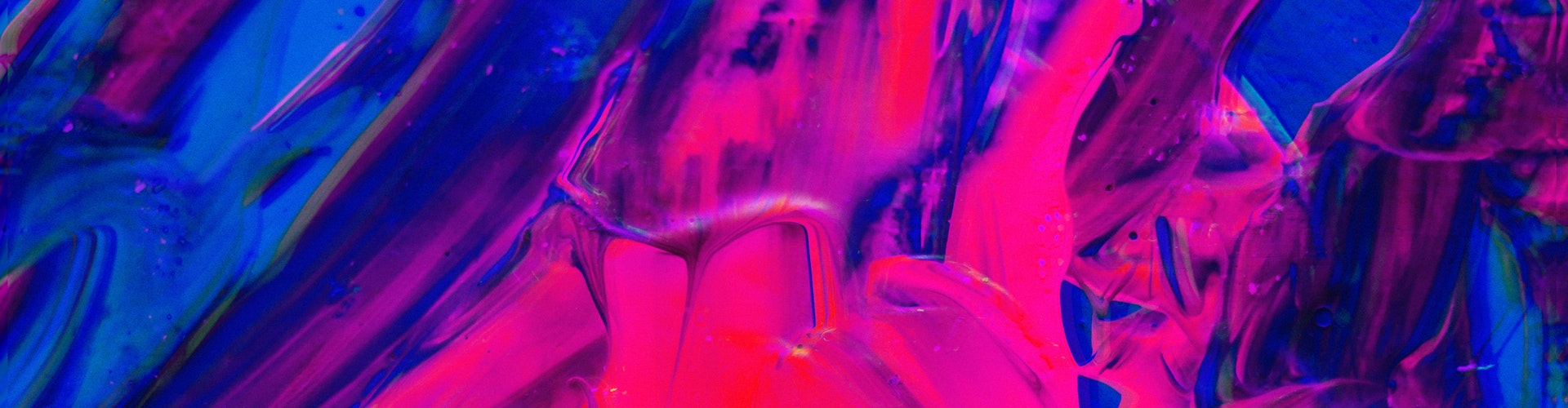
Running a Flask backend and Next.js together
Described is a common setup for modern web applications. Flask handles the backend logic, while Next.js serves as the frontend framework.
Here’s a step-by-step guide to set up and run both together:
1. Project Structure
Organize your project into two main folders: one for the Flask backend and one for the Next.js frontend.
my-app/
├── backend/
│ ├── app.py
│ ├── requirements.txt
│ └── ...
├── frontend/
│ ├── pages/
│ ├── public/
│ ├── package.json
│ └── ...
└── docker-compose.yml (optional for containerization)
2. Set Up Flask Backend
Step 1: Create a Flask App
Create a simple Flask app in the backend
folder.
# backend/app.py
from flask import Flask, jsonify
app = Flask(__name__)
@app.route('/api/hello')
def hello():
return jsonify(message="Hello from Flask!")
if __name__ == '__main__':
app.run(host='0.0.0.0', port=5000)
Step 2: Install Flask Dependencies
Create a requirements.txt
file in the backend
folder and install the dependencies.
# backend/requirements.txt
Flask==2.3.2
Run:
pip install -r requirements.txt
Step 3: Run Flask
Run the Flask app:
python app.py
The Flask backend will now be running on http://localhost:5000
.
3. Set Up Next.js Frontend
Step 1: Create a Next.js App
Navigate to the frontend
folder and create a new Next.js app using create-next-app
.
npx create-next-app@latest frontend
cd frontend
Step 2: Modify Next.js to Call Flask API
In your Next.js app, you can call the Flask API using fetch
or axios
.
For example, modify the pages/index.js
file:
// frontend/pages/index.js
import { useEffect, useState } from 'react';
export default function Home() {
const [message, setMessage] = useState('');
useEffect(() => {
// Fetch data from Flask backend
fetch('http://localhost:5000/api/hello')
.then((response) => response.json())
.then((data) => setMessage(data.message));
}, []);
return (
<div>
<h1>Next.js Frontend</h1>
<p>Message from Flask: {message}</p>
</div>
);
}
Step 3: Run Next.js
Run the Next.js development server:
npm run dev
The Next.js frontend will now be running on http://localhost:3000
.
4. Proxy Requests (Optional)
If you want to avoid CORS issues during development, you can set up a proxy in the Next.js app.
Step 1: Add Proxy to next.config.js
Modify the next.config.js
file to proxy API requests to the Flask backend.
// frontend/next.config.js
module.exports = {
async rewrites() {
return [
{
source: '/api/:path*',
destination: 'http://localhost:5000/api/:path*', // Proxy to Flask backend
},
];
},
};
Step 2: Update API Calls
Update your API calls in the Next.js app to use the proxied path:
fetch('/api/hello') // This will be proxied to Flask
5. Run Both Together
You can run both the Flask backend and Next.js frontend simultaneously. Use two terminal windows:
Terminal 1: Run Flask
cd backend python app.py
Terminal 2: Run Next.js
cd frontend npm run dev
6. Containerize with Docker (Optional)
If you want to containerize both the Flask backend and Next.js frontend, you can use Docker and Docker Compose.
Step 1: Create Dockerfiles
Flask Dockerfile:
# backend/Dockerfile FROM python:3.9-slim WORKDIR /app COPY requirements.txt . RUN pip install -r requirements.txt COPY . . CMD ["python", "app.py"]
Next.js Dockerfile:
# frontend/Dockerfile FROM node:16 WORKDIR /app COPY package.json package-lock.json ./ RUN npm install COPY . . CMD ["npm", "run", "dev"]
Step 2: Create docker-compose.yml
version: '3.8'
services:
backend:
build: ./backend
ports:
- "5000:5000"
networks:
- app-network
frontend:
build: ./frontend
ports:
- "3000:3000"
networks:
- app-network
networks:
app-network:
driver: bridge
Step 3: Run Docker Compose
Run both services with Docker Compose:
docker-compose up
7. Production Deployment
For production, you can:
- Build the Next.js app:
npm run build npm start
- Deploy the Flask backend and Next.js frontend separately (e.g., on AWS, Heroku, or Vercel).
Summary
- Flask runs on
http://localhost:5000
and serves the backend API. - Next.js runs on
http://localhost:3000
and consumes the Flask API. - Use a proxy in development to avoid CORS issues.
- Optionally, containerize both apps using Docker and Docker Compose.
This setup allows you to develop and run a Flask backend and Next.js frontend together seamlessly!